Introduction
- Explain what HTTP methods are and their role in web communication.
- Define HTTP and how request methods fit into the request-response model.
Overview of Common Methods
- GET: Retrieves data from a server.
- Example: Fetching user details from `/users/123`.
- POST: Submits data to be processed to a server.
- Example: Submitting a form to `/users`.
- PUT: Updates or creates a resource at a specified URL.
- Example: Updating user information at `/users/123`.
- DELETE: Removes a resource from a server.
- Example: Deleting a user at `/users/123`.
- PATCH: Partially updates a resource.
- Example: Updating user email at `/users/123`.
Use Cases
- Provide scenarios where each method is appropriate.
- Discuss best practices for choosing the right method.
Conclusion
- Recap the importance of understanding these methods for effective web development.
2. Understanding HTTP GET vs. POST: When to Use Each
Introduction
- Briefly explain GET and POST methods and their fundamental differences.
GET Method
- Purpose: Fetch data from the server.
- Characteristics:
- Safe and idempotent (doesn't alter server state).
- Data is sent in the URL.
- Limited data size.
- Use Case Examples:
- Fetching user profiles, search results.
POST Method
- Purpose: Submit data to be processed by the server.
- Characteristics:
- Not idempotent (multiple submissions can have different effects).
- Data is sent in the request body.
- Suitable for large amounts of data.
- Use Case Examples:
- Submitting forms, creating new records.
Comparison
- Discuss performance considerations, security implications, and data handling.
Conclusion
- Summarize when to use GET and POST based on specific needs and scenarios.
3. The Role of HTTP PUT and PATCH in API Design
Introduction
- Explain PUT and PATCH methods and their role in updating resources.
PUT Method
- Purpose: Replace or create a resource at a specific URL.
- Characteristics:
- Idempotent (repeated requests produce the same result).
- Requires the complete resource representation.
- Use Case Examples:
- Updating a user profile with a complete new set of data.
PATCH Method
- Purpose: Apply partial modifications to a resource.
- Characteristics:
- Not necessarily idempotent.
- Requires only the changes to the resource.
- Use Case Examples:
- Updating a single field like email address or status.
Design Considerations
- Discuss when to use PUT vs. PATCH.
- Provide best practices for implementing these methods in RESTful APIs.
Conclusion
- Recap the importance of choosing the right update method for efficient and accurate resource management.
4. HTTP DELETE: Safely Removing Resources from Your Application
Introduction
- Explain the purpose and importance of the DELETE method.
DELETE Method
- Purpose: Remove a resource from the server.
- Characteristics:
- Idempotent (deleting the same resource multiple times has the same effect).
- Can include considerations for soft deletes versus hard deletes.
Use Case Examples
- Deleting a user account.
- Removing items from a shopping cart.
Implementation Considerations
- Handling resource dependencies and potential side effects.
- Ensuring proper authorization and validation before deletion.
Conclusion
- Summarize best practices for implementing safe and effective DELETE operations.
5. Exploring Lesser-Known HTTP Methods: OPTIONS, HEAD, and TRACE
Introduction
- Briefly introduce these less common HTTP methods.
OPTIONS Method
- Purpose: Describe the communication options for a resource.
- Characteristics:
- Useful for CORS preflight requests.
- Provides allowed methods and other options.
HEAD Method
- Purpose: Similar to GET but retrieves only headers, not the body.
- Characteristics:
- Useful for checking resource metadata or existence.
TRACE Method
- Purpose: Echoes back the received request for diagnostic purposes.
- Characteristics:
- Rarely used in production due to security concerns.
Use Cases and Considerations
- Provide examples and scenarios where each method might be used.
- Discuss potential security risks and best practices.
Conclusion
- Recap the utility and considerations of using these lesser-known methods.
6. The Impact of HTTP Request Methods on Web Security
Introduction
- Explain the relevance of HTTP methods to web security.
Security Implications of GET and POST
- Discuss common vulnerabilities like XSS and CSRF.
- Strategies to secure GET and POST requests.
Security Implications of PUT, PATCH, DELETE
- Risks associated with resource modification and deletion.
- Importance of validation, authorization, and logging.
Mitigation Strategies
- Implementing secure practices, such as input validation and proper authentication mechanisms.
Conclusion
- Summarize key takeaways for maintaining secure HTTP methods.
7. How HTTP Request Methods Affect Performance and Scalability
Introduction
- Explain how HTTP methods can impact application performance.
Performance Considerations
- GET: Efficient but may become a bottleneck with high traffic or large payloads.
- POST: Can impact server performance due to data processing.
- PUT/PATCH: Resource updates can affect database performance and cache invalidation.
Scalability Challenges
- Discuss how different methods affect server load and scaling strategies.
- Considerations for optimizing performance, like caching strategies and request batching.
Conclusion
- Summarize best practices for handling performance and scalability issues related to HTTP methods.
8. Best Practices for Using HTTP Request Methods in RESTful APIs
Introduction
- Define RESTful API principles and the role of HTTP methods.
Best Practices
- Naming Conventions: Use consistent, descriptive naming for endpoints.
- Idempotency: Ensure methods like PUT and DELETE are idempotent where applicable.
- Error Handling: Standardize error responses for each method.
- Documentation: Clearly document API methods and their expected behaviors.
Case Studies
- Provide examples of well-designed RESTful APIs and how they implement best practices.
Conclusion
- Recap the importance of following best practices for a maintainable and user-friendly API.
9. HTTP Request Methods and Their Role in Web Services and Microservices
Introduction
- Explain the role of HTTP methods in service-oriented architectures.
Web Services
- Discuss how methods are used in SOAP and RESTful services.
Microservices
- Service Communication: How methods facilitate communication between microservices.
- Data Consistency: Handling data consistency and synchronization across services.
Case Studies
- Provide examples of how HTTP methods are utilized in real-world microservices architectures.
Conclusion
- Summarize how understanding HTTP methods aids in designing effective web and microservices.
10. Debugging and Testing HTTP Request Methods: Tools and Techniques
Introduction
- Explain the importance of debugging and testing HTTP requests.
Tools
- Postman: Features for testing and automating HTTP requests.
- cURL: Command-line tool for making HTTP requests.
- Browser Developer Tools: Network panel for monitoring requests and responses.
Techniques
- Unit Testing: Writing tests for API endpoints.
- Mocking Services: Simulating server responses for testing.
- Debugging: Analyzing and troubleshooting issues in requests and responses.
Best Practices
- Tips for effective debugging and ensuring reliable tests.
Conclusion
- Recap the importance of thorough testing and debugging for robust API development.
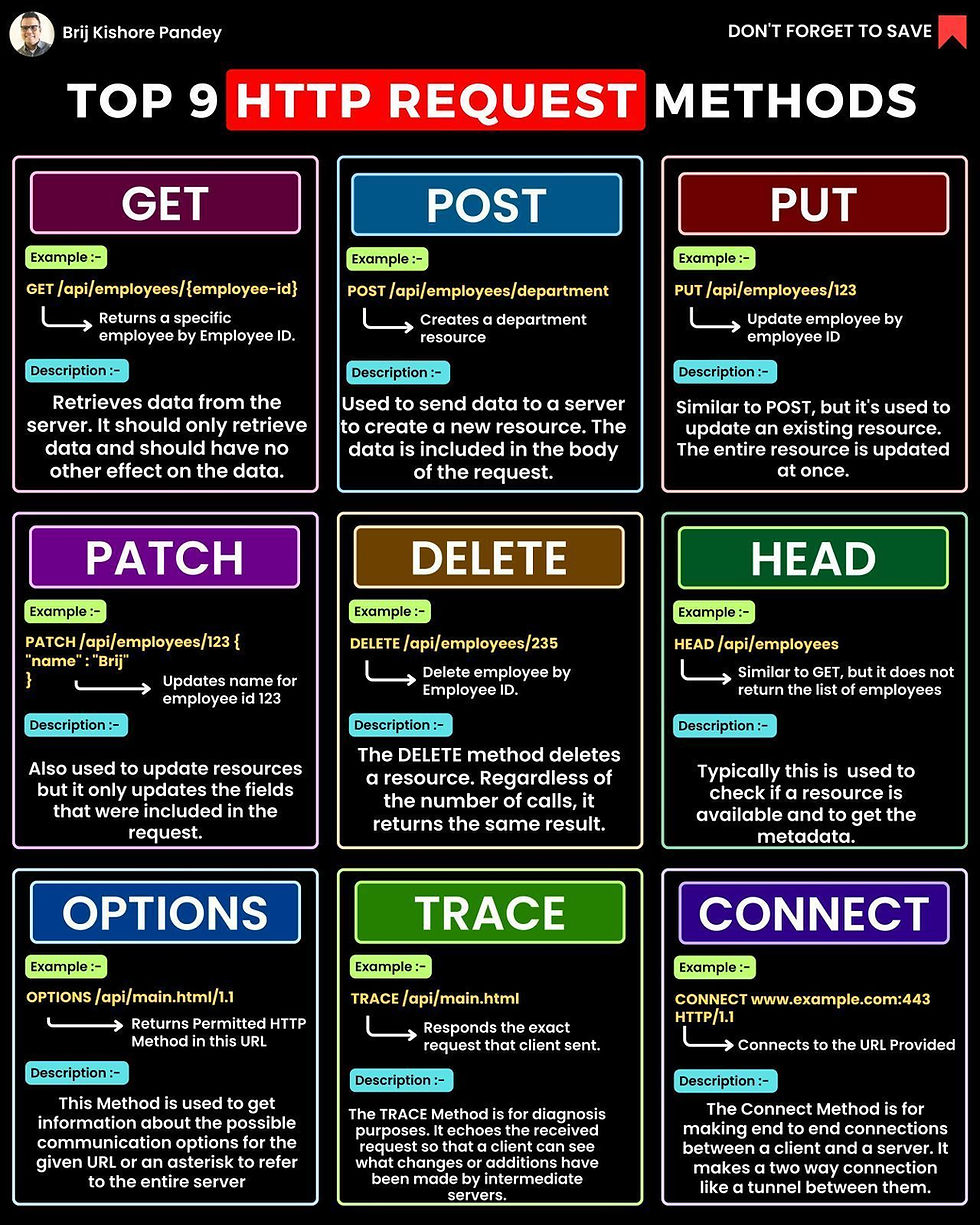
コメント